WholeSlideAnnotation#
from wholeslidedata import WholeSlideAnnotation
from wholeslidedata.visualization.plotting import plot_annotations
from colour import Color
from matplotlib import pyplot as plt
wsa = WholeSlideAnnotation('/tmp/TCGA-21-5784-01Z-00-DX1.xml')
print(len(wsa.annotations))
print(wsa.labels.names)
color_map = {label_name: Color(pick_for=label_name).hex_l for label_name in wsa.labels.names}
10
['lymphocytes', 'stroma', 'tumor']
plot_annotations(wsa.annotations, color_map=color_map)
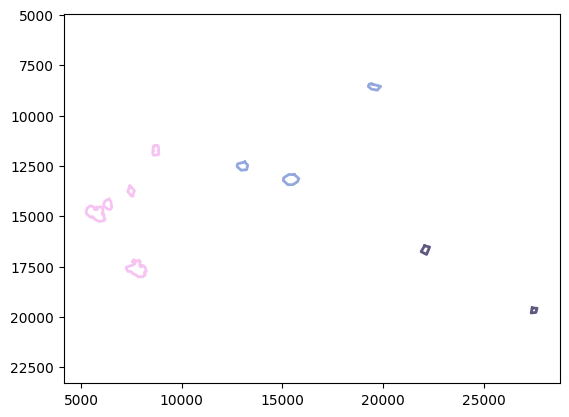
selected_annotations = wsa.select_annotations(center_x=7500, center_y=15000, width=5000, height=10000)
lymphocyte_annotations = [annotation for annotation in wsa.annotations if annotation.label.name == "lymphocytes"]
fig, axes = plt.subplots(1,2)
plot_annotations(selected_annotations, ax=axes[0], color_map=color_map)
plot_annotations(lymphocyte_annotations, ax=axes[1], color_map=color_map)
plt.show()
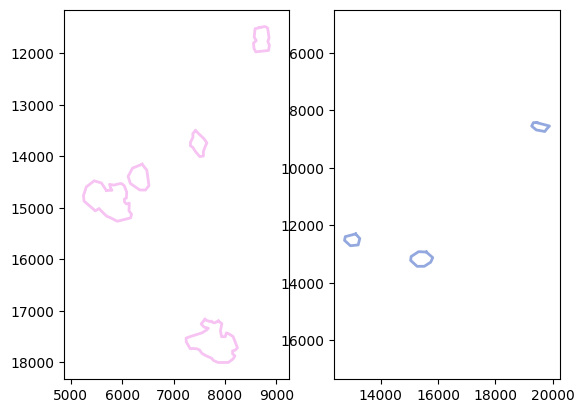
Label names#
wsa = WholeSlideAnnotation('/tmp/TCGA-21-5784-01Z-00-DX1.xml', labels={'tumor':1, 'stroma': 2, 'lymphocytes':2}, renamed_labels={'tumor':1, 'other':2})
print(wsa.labels.names)
color_map = {label_name: Color(pick_for=label_name).hex_l for label_name in wsa.labels.names}
plot_annotations(wsa.annotations, color_map=color_map)
['other', 'tumor']
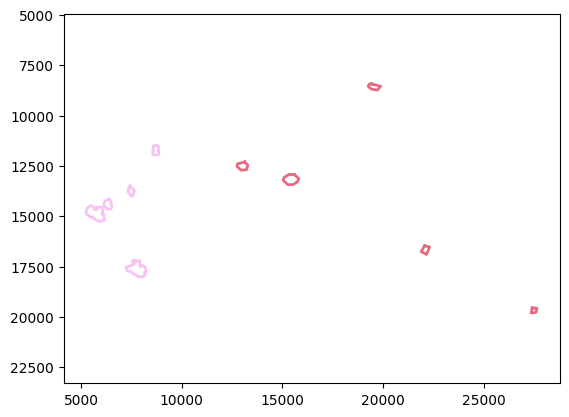
Callbacks#
from wholeslidedata.annotation.hooks import ScalingAnnotationHook, TiledAnnotationHook
wsa = WholeSlideAnnotation('/tmp/TCGA-21-5784-01Z-00-DX1.xml', hooks=(ScalingAnnotationHook(0.5),))
color_map = {label_name: Color(pick_for=label_name).hex_l for label_name in wsa.labels.names}
plot_annotations(wsa.annotations, color_map=color_map)
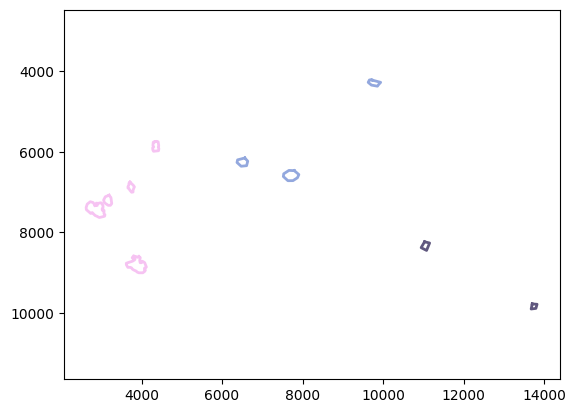
labels={'tumor':1, 'stroma': 2, 'lymphocytes':2}
wsa = WholeSlideAnnotation('/tmp/TCGA-21-5784-01Z-00-DX1.xml', labels=labels, hooks=(TiledAnnotationHook(tile_size=256, label_names=list(labels)),))
color_map = {label_name: Color(pick_for=label_name).hex_l for label_name in wsa.labels.names}
plot_annotations(wsa.annotations, color_map=color_map)
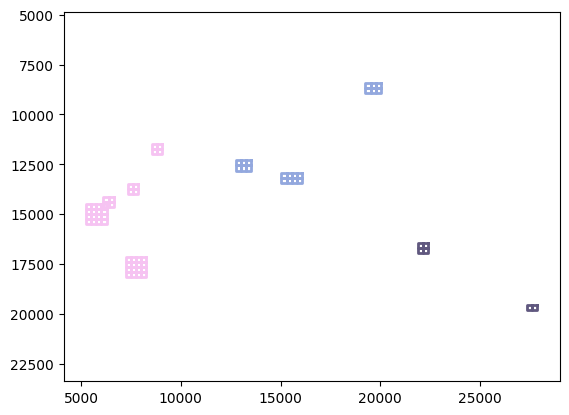
selected_annotations = wsa.select_annotations(center_x=7500, center_y=15000, width=5000, height=10000)
plot_annotations(selected_annotations, color_map=color_map)
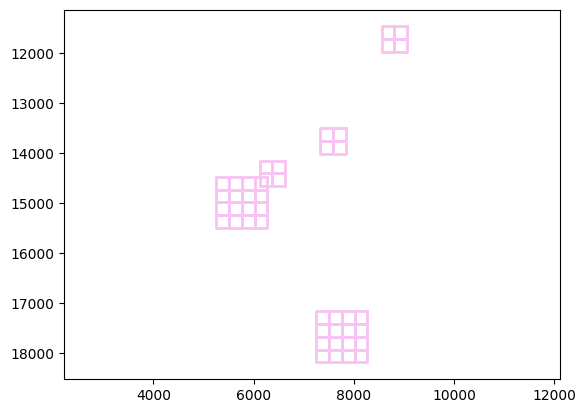